How to calculate VAT | Add or Exclude VAT on Certain Amount 💶
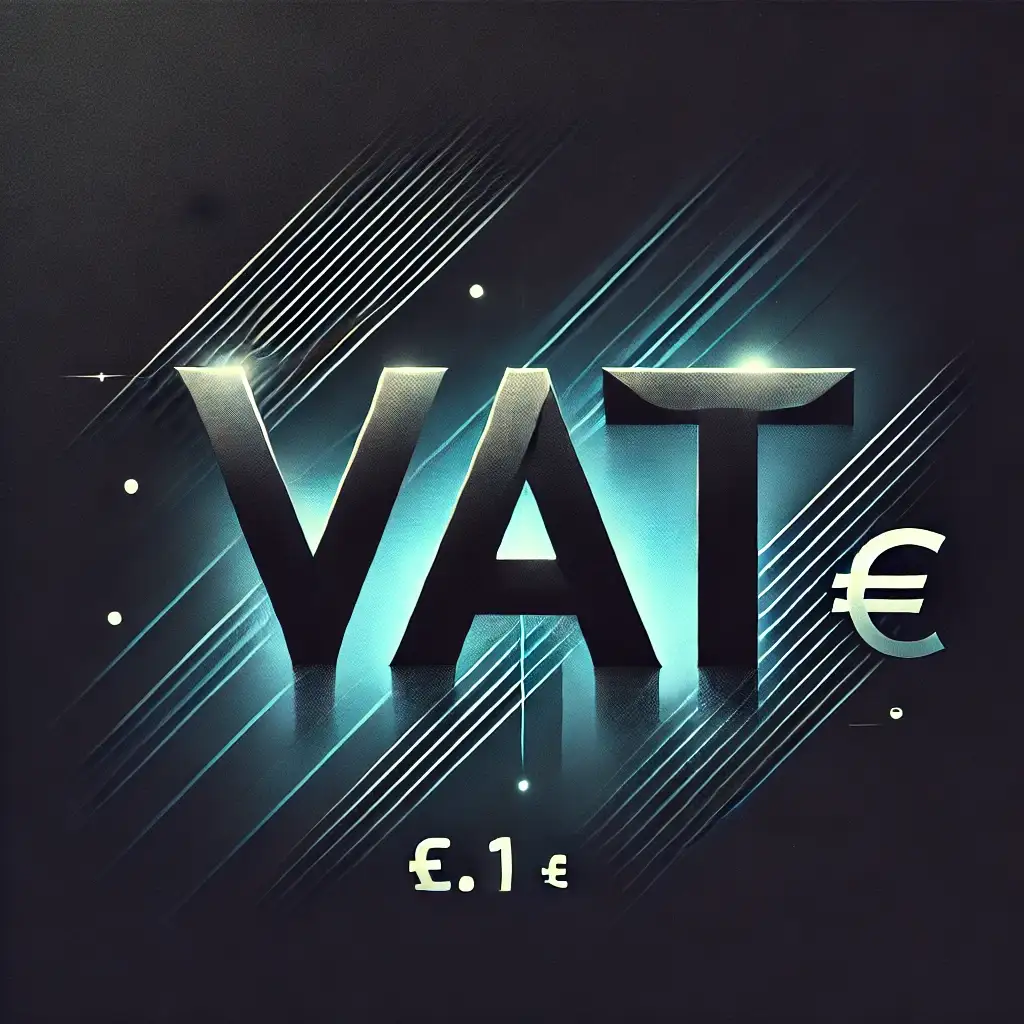
VAT Calculation: Add or Exclude VAT on a Certain Amount.
How to calculate VAT in simple wording. In this article, I use PHP to perform VAT calculations. How to calculate VAT in PHP. I also provide VAT_Trait.php
below so, you can use that trait in any PHP project or frameworks like Laravel, Laminas, Zend or CakePHP, etc.
Using the above example you can calculate VAT on x-amount. Add VAT on x-amount or Exclude VAT on x-amount. And also calculate the added or excluded VAT value on the amount.
Requirements
you need VAT Percentage. current VAT percentage is 20% means In this article I’ll calculate VAT on X-Amount
using 20% VAT
.
PHP 8.0
, column decimal(12, 2)
Let’s Understand first
20% VAT add on 100 amount is = 120
20% VAT excluded on 100 amount is = 83.33
In-Depth
20% VAT add on 100 amount is = 120
Amount | VAT, % | Operation | VAT added | Gross amount |
100 | 20 | add | 20.00 | 120.00 |
20% VAT excluded on 100 amount is = 83.33
Amount | VAT, % | Operation | VAT excluded | Net amount |
100 | 20.00 | exclude | 16.67 | 83.33 |
Calculation
<?php
const VAT_PERCENTAGE = 20; // 20%
class Cart
{
public function calcAddVatToAmount($vat_percentage, $amount): float|int
{
// $amount * (1 + $vat_percentage / 100)
return round($amount * (1 + $vat_percentage / 100), 2); // simple roundof
//or simple
/*
* calculate 20% tax on x-amount
* ((20 % 100) * x-amount) + x-amount
* */
}
$cart = new Cart();
echo $cart->calcAddVatToAmount(VAT_PERCENTAGE, 9);
// Gross amount: 10.80
public function calcExcludeVatFromAmount($vat_percentage, $amount): float|int
{
// $amount - ($amount - $amount / (1 + $vat_percentage / 100)
return round($amount - ($amount - $amount / (1 + $vat_percentage / 100)), 2);
}
$cart = new Cart();
echo $cart->calcExcludeVatFromAmount(VAT_PERCENTAGE, 9);
// Net amount: 7.50
// if you want to check how much VAT is added on x-amount
public function calcVatAddedValue($vat_percentage, $amount): float
{
$amount_including_vat = $this->calcAddVatToAmount($vat_percentage, $amount);
// $amount_including_vat - $amount
return round($amount_including_vat - $amount, 2); // simple roundof
}
$cart = new Cart();
echo $cart->calcVatAddedValue(VAT_PERCENTAGE, 9);
// VAT added: 1.80
// How much VAT value is excluded then
public function calcVatExcludedValue($vat_percentage, $amount): float|int
{
// $amount - $amount / (1 + $vat_percentage / 100)
return round($amount - $amount / (1 + $vat_percentage / 100), 2);
}
$cart = new Cart();
echo $cart->calcVatExcludedValue(VAT_PERCENTAGE, 9);
// VAT excluded: 1.50
}
Step-by-step VAT functions
Let’s destructure Cart Class.
Calculate VAT Add on amount
<?php
const VAT_PERCENTAGE = 20; // 20%
class Cart
{
public function calcAddVatToAmount($vat_percentage, $amount): float|int
{
return round($amount * (1 + $vat_percentage / 100), 2);
}
}
$cart = new Cart();
echo $cart->calcAddVatToAmount(VAT_PERCENTAGE, 9);
// Gross amount: 10.80
Calculate VAT Exclude on amount
<?php
const VAT_PERCENTAGE = 20; // 20%
class Cart
{
public function calcExcludeVatFromAmount($vat_percentage, $amount): float|int
{
return round($amount - ($amount - $amount / (1 + $vat_percentage / 100)), 2);
}
}
$cart = new Cart();
echo $cart->calcExcludeVatFromAmount(VAT_PERCENTAGE, 9);
// Net amount: 7.50
Calculate how must VAT added to the amount
<?php
const VAT_PERCENTAGE = 20; // 20%
class Cart
{
public function calcAddVatToAmount($vat_percentage, $amount): float|int
{
return round($amount * (1 + $vat_percentage / 100), 2);
}
public function calcVatAddedValue($vat_percentage, $amount): float
{
$amount_including_vat = $this->calcAddVatToAmount($vat_percentage, $amount);
return round($amount_including_vat - $amount, 2);
}
}
$cart = new Cart();
echo $cart->calcVatAddedValue(VAT_PERCENTAGE, 9);
// VAT added: 1.80
Calculate how must VAT excluded from the amount
<?php
const VAT_PERCENTAGE = 20; // 20%
class Cart
{
public function calcVatExcludedValue($vat_percentage, $amount): float|int
{
return round($amount - $amount / (1 + $vat_percentage / 100), 2);
}
}
$cart = new Cart();
echo $cart->calcVatExcludedValue(VAT_PERCENTAGE, 9);
// VAT excluded: 1.50
VAT Trait for PHP projects e.g Laravel, Laminas, etc
You can use the below trait in any PHP project.
Download file Github Gist
Embedded File
Raw File
<?php
namespace App\Helpers;
class VAT_Helper
{
const VAT_PERCENTAGE = 20;
}
// -------------------------------------
?>
<?php
namespace App\Traits;
use App\Helpers\VAT_Helper;
trait VAT_Trait
{
public function calcAddVatToAmount(float $amount, int $vat_percentage = VAT_Helper::VAT_PERCENTAGE): float|int
{
return round($amount * (1 + $vat_percentage / 100), 2);
// e.g Gross amount: 10.80
}
public function calcVatAddedValue(float $amount, int $vat_percentage = VAT_Helper::VAT_PERCENTAGE): float
{
$amount_including_vat = $this->calcAddVatToAmount($amount, $vat_percentage);
return round($amount_including_vat - $amount, 2);
// e.g VAT added: 1.80
}
public function calcExcludeVatFromAmount(float $amount, int $vat_percentage = VAT_Helper::VAT_PERCENTAGE): float|int
{
return round($amount - $amount / (1 + $vat_percentage / 100), 2);
// e.g Net amount: 7.50
}
public function calcVatExcludedValue(float $amount, int $vat_percentage = VAT_Helper::VAT_PERCENTAGE): float|int
{
return round($amount - $amount / (1 + $vat_percentage / 100), 2);
// e.g VAT excluded: 1.50
}
}
// ---------------------------------------------------
?>
<!-- How to use -->
<?php
namespace App\Http\Services;
use App\Helpers\VAT_Helper;
class CartService
{
use VAT_Trait;
const VAT_PERCENTAGE = 20; // you can skip this because default VAT percentage added i VAT_Trait.
public function createCartItem(Request $request)
{
$product_price = $request->input('product_price'); // e.g 9$
$cartItem = CartItem::create([
'product_id' => $request->input('product_id'),
'sku' => $request->input('sku'),
'total_weight' => $request->input('weight') * $request->input('quantity'),
// single amount
'price' => (float) $this->calcAddVatToAmount($product_price, VAT_Helper::VAT_PERCENTAGE), // 2nd parameter is optional
// single amount * quantity
'total' => (float) $this->calcAddVatToAmount($product_price * 5, VAT_Helper::VAT_PERCENTAGE),
// check how much VAT added on single amount
'tax_amount' => (float) $this->calcVatAddedValue($product_price, VAT_Helper::VAT_PERCENTAGE),
// check how much VAT added on (single amount * quantity)
'total_tax_amount' => (float) $this->calcVatAddedValue($product_price * 5, VAT_Helper::VAT_PERCENTAGE),
]);
}
}
?>
Summary
Using the above example you can calculate VAT on x-amount. Add VAT on x-amount or Exclude VAT on x-amount. And also calculate the added or excluded VAT value on the amount.